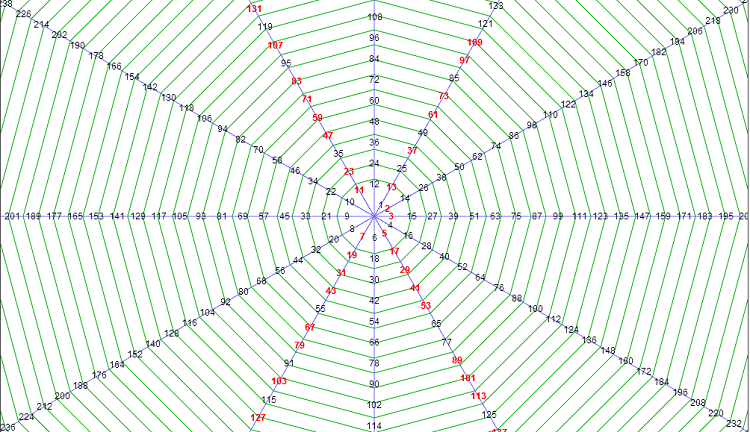
12-Spiral in HTML Canvas
(Primes highlighted in red)
Mathematics is about exploration, not the rote memorization of algortithms. It’s about finding patterns, appreciating how numbers relate to one another. That’s why I love programming. Much of writing code involves experimentation with mathematics, tweeking a variable to see what comes out of a complex function. It’s wonderful when a program doesn’t give you the answer you designed it to; that means you are about to learn something.
I was really inspired by this post on Moebius Noodles about Joey Grether, who creates spiraling artwork with the number 12 that seeks patterns in the lines of numbers radiating out from the center. Reminiscent of Ulam’s Spiral, building a spiral around a clock, with 12-segments in the rotation, puts multiples of 3 at {3,6,9,12} o’clock, multiples of 4 at {4,8,12} o’clock, multiples of 2 at {2,4,6,8,10,12} o’clock, and primes at {1,5,7,11}.
Knowing that programmers had written Ulam’s and Sack’s Spiral Generators, I wondered if I could do the same with this 12 spiral. In a few hours I was reacquainted with many mathematical concepts, from degrees, to radians, to algorithms for finding primes and their optimization. It’s a great, zenlike feeling to be immersed in the beauty of logic, and, in the interest of sharing that feeling, I decided to write a sort of code-essay to encourage others to download my rough draft, play with it, and maybe even take it further.
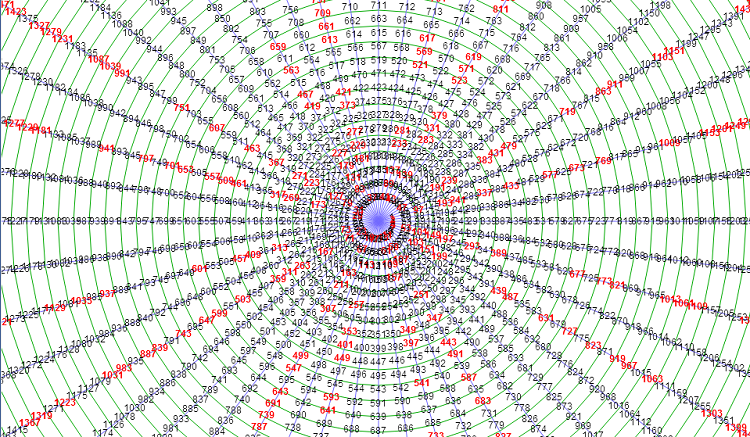
48-Spiral in HTML Canvas
(Primes highlighted in red)
Playing around with this code, I found there’s a symmetry to the spirals that are multiples of 12 {24, 36, 48, 60} that I couldn’t find in other spirals. While {12, 24, 48, 96…} appear to have a mirror symmetry along the left and right, other factors of 12, like 60, have a different kind of symmetry that I don’t quite know how to describe. The number 12 remains my favorite number for it’s simplicity, symmetry, and what it reveals about the relationships between numbers, seeming to categorize them along the spiral branches.
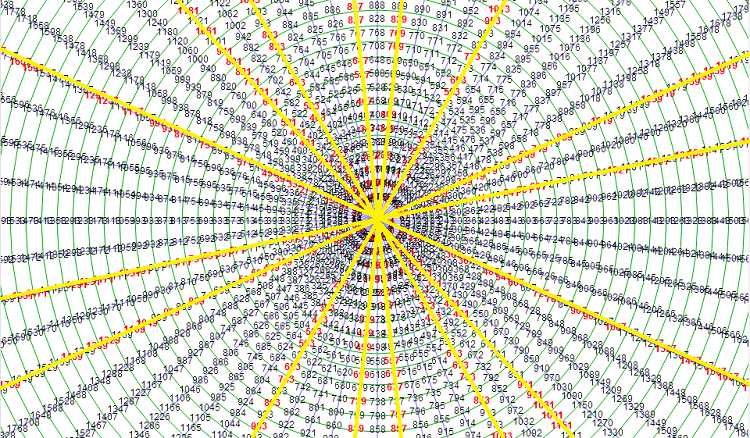
60-Spiral in HTML Canvas
(Primes highlighted in red, symmetry highlighted yellow)
You don’t need any special environment to run this code on your computer, simply Right-Click –>HERE and select “Save As” to save the html file to your computer, then drag-and-drop it into your browser window or double-click on it to launch it. You can then edit it in notepad or, my favorite, the free Sublime Text Editor. Edit the code, save, and then refresh your browser to see the result. Super simple.
[/ccie_javascript]